Use Python unittest to Master Test-Driven Development
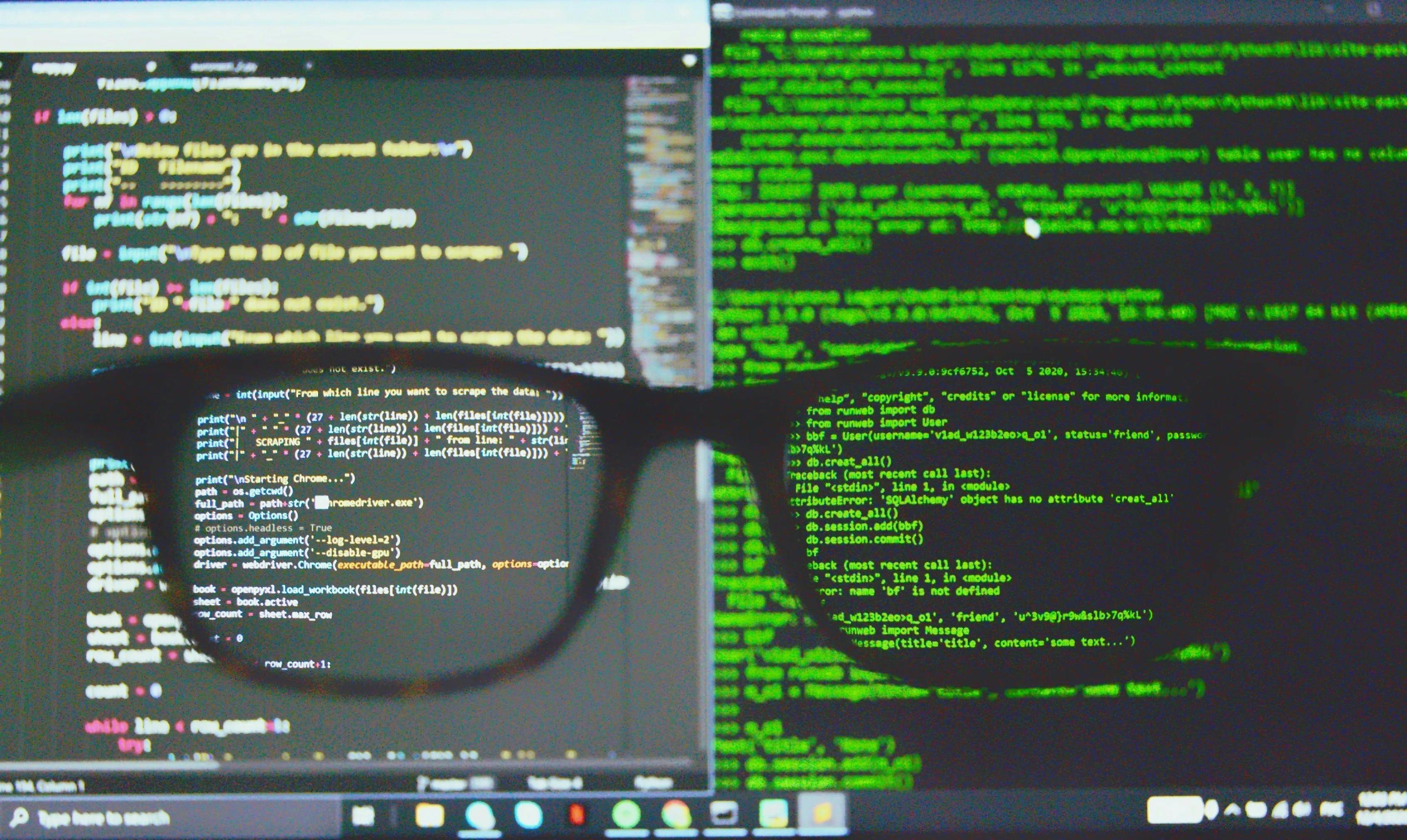
By Avalith Editorial Team ♦ 1 min read
What Are Unit Tests in Python?
If you want to understand unit testing in Python specifically, you’re in the right place.
First things first: unit testing is a method for testing software that works with units, or the smallest testable pieces of a code. When we say testing, we’re talking about making sure that these units are operating correctly, so unit testing is essentially verifying that each little piece of the code works correctly. Sometimes, we need to make changes to our code and running unit tests makes sure that these changes haven’t impacted functionality.
Unit testing also helps isolate errors by looking at the outputs and seeing where in our code the bug or bugs are and going from there. Even though unit testing doesn’t always solve the problems, it definitely helps and is where you should begin before heading into integration testing.
Test Driven Development
Test driven development, or TDD, is a software development process based on testing. Software development should be based around a set of unit tests, making it the basis of the entire development process. Each component that you develop along the way should have a corresponding test. TDD also leans towards testing fewer components at a time and running more specific tests, helping to isolate errors.
The Assert Statement and the Python Unittest Module
Python has a built-in statement called assert which is used to see whether given conditions are true or not. If it is, nothing happens, but if it isn’t, an error is raised. Asserts are used solely for debugging and testing reasons, not for handling the errors themselves.
The unittest module works with object-oriented concepts. If you’re new to Python, you’ll need to get up to speed with some of the basics, including classes and methods in this specific language. Unittest provides us with multiple tools to help test code, and one of the most important classes is the test case, used as a base class to help us run several tests simultaneously. The good thing about this class is that it already works like the assert statement, meaning it provides its own assert methods like the assertEqual and the assertTrue (to test and see whether elements are equal or true or false, respectively).
The Python unittest module allows developers to create a test case class that gets its information from unitest.TestCase class. You’ll use this to create some code for testing. Within this class, test methods are defined and named with the prefix “test_” to be easily discoverable within the testing framework.
The Python module gives developers several benefits including:
Built-in Testing Framework: unittest is built into Python, so you won’t need added installations. You can easily access it and use it across different Python environments.
Simple and Familiar Syntax: Like we’ve seen, the unittest framework uses a class-based approach. Test cases are classes that inherit from unittest.TestCase which are intuitive and are already familiar to developers.
Assertion Methods: unittest providesseveral assertion methods to compare expected and actual results.
Test Discovery: Automated test discovery enables the discovery and execution of all test cases within a directory or module.
Test Fixtures: You can define setup and teardown methods within test cases. Methods like setUp() and tearDown() let you set up the environment you need before executing each test and clean up resources once you’re done.
Test Suites: unittest supports test suite creation.
Run Unittest in Python
If you want to try running unit tests in Python, you need to isolate some code to test or write code to test it out as an example. Write a set_name() function to store your data and the get_name() function to retrieve it. You can now create an individual test case by subclassing unittest.TestCase, and you can add logic to the test by overriding or adding functions.
The unittest function has several outcomes and functions:
The assertEqual(a,b) checks that a==b
The assertNotEqual(a,b) checks that a != b
The assertTrue(x) checks that bool(x) is True
The assertFalse(x) checks that bool(x) is False
The assertIs(a,b) checks that a is b
The assertIsNot(a, b) checks that a is not b
The assertIsNone(x) checks that x is None
The assertIsNotNone(x) checks that x is not None
The assertIn(a, b) checks that a in b
The assertNotIn(a, b) checks that a not in b
The assertIsInstance(a, b) checks that isinstance(a, b)
The assertNotIsInstance(a, b) checks that not isinstance(a, b)
You can run these unit tests within integrated developer environments (or IDEs) like PyCharm or Visual Studio. These make it more convenient to work with larger projects that include several files.
Differences Between PyTest and Unittest
Let’s take a look at some of the features of PyTest and Unittest and see where the differences lie:
Test Discovery: Pytest allows for automatic test discovery, and can find and run tests without boilerplate, while Unittest requires manual discovery and explicitly defining test cases
Fixture Support: Pytest has powerful, flexible fixture support while Unittest offers limited fixture support through setup and teardown methods
Test Execution: Pytest supports parallel test execution and a faster runtime while Unittest supports a sequential test execution, meaning you can run one test at a time
Test Execution Options: Pytest provides options for test execution customization while Unittest offers fewer options for customizing your testing
Assertion Methods: Pytest has a series of built-in assertion methods while Unittest has the standard assertion methods provided by the unittest module
Test Organization: Python’s test functions can be organized in different ways, while Unittest cases are organized as classes, providing a more structured approach
Skipping Tests: Pytest has a built-in mechanism for skipping tests, while Unittest lets you skip using decorators or conditional statements
Test Parameterization: With PyTest, you get built-in support for parameterized tests but parameterization can be achieved using decorators or conditional logic with Unittest
Plugin Ecosystem: PyTest boasts a large and active plugin ecosystem, Unittest has limited plugin support, fewer third-party extensions, etc.
Output Readability: PyTest provides you with detailed and readable output for failed tests, Unittest provides a basic output with less details
Integration with IDEs: PyTest integrates well with various IDEs, plugins, and reporting tools but Unittest provides basic integration
Python Version Support: PyTest is compatible with Python 2.7 and above, Unittest is compatible with Python 2.1 and above
Running tests to detect bugs on time is important for every developer not only because they’ll be able to fix the errors eventually but also because it saves time in the process. Badly written code with glitches can be costly, time consuming and a hindrance to the programming process and to an organization’s functionality. Testing for bugs is always good practice when you’re looking to maintain clean code, which is key when you need things to work properly. Bad code can function, but clean code keeps everything running smoothly.